Script Examples for Specific Functions
GetApplicationPath
Copy and paste the script below and monitor the 'Script output' to see the result.
@scriptname="Example GetApplicationPath";
@author="";
@version="MADRIX 2.8a";
@description="Display the application path,
running from script engine version 1.25 and MADRIX 2.8a";
void InitEffect()
{
}
void PreRenderEffect()
{
}
void PostRenderEffect()
{
WriteText(GetApplicationPath());
}
(Description)
GetUserProfileDirectory
Copy and paste the script below and monitor the 'Script output' to see the result.
@scriptname="Example GetUserProfileDirectory";
@author="";
@version="MADRIX 2.8a";
@description="Display the user profile directory,
running from script engine version 1.25 and MADRIX 2.8a";
void InitEffect()
{
}
void PreRenderEffect()
{
}
void PostRenderEffect()
{
WriteText(GetUserProfileDirectory());
}
(Description)
CheckScriptEngineVersion
Copy and paste the script below and monitor the 'Script output' to see the result.
@scriptname="Example CheckScriptEngineVersion";
@author="";
@version="MADRIX 2.8a";
@description="Check script engine version number,
running from script engine version 1.25 and MADRIX 2.8a";
void InitEffect()
{}
void PreRenderEffect()
{}
void PostRenderEffect()
{
if(CheckScriptEngineVersion(1,25)>0)
WriteText("Script engine version ok");
else
WriteText("Script engine version too old");
}
(Description)
CheckSoftwareVersion
Copy and paste the script below and monitor the 'Script output' to see the result.
@scriptname="Example CheckSoftwareVersion";
@author="";
@version="MADRIX 2.8a";
@description="Check software version number,
running from script engine version 1.25 and MADRIX 2.8a";
void InitEffect()
{}
void PreRenderEffect()
{}
void PostRenderEffect()
{
if(CheckSoftwareVersion(2,8,1,0)>0)
WriteText("Madrix version ok");
else
WriteText("Madrix version too old");
}
(Description)
GetScriptEngineVersion
Copy and paste the script below and monitor the 'Script output' to see the result.
@scriptname="Example GetScriptEngineVersion";
@author="";
@version="MADRIX 2.8a";
@description="Get MADRIX version,
running from script engine version 1.25 and MADRIX 2.8a";
void InitEffect()
{}
void PreRenderEffect()
{}
void PostRenderEffect()
{
WriteText("ScriptEngine " +GetScriptEngineVersion());
}
(Description)
GetSoftwareVersion
Copy and paste the script below and monitor the 'Script output' to see the result.
@scriptname="Example GetSoftwareVersion";
@author="";
@version="MADRIX 2.8a";
@description="Get MADRIX version,
running from script engine version 1.25 and MADRIX 2.8a";
void InitEffect()
{}
void PreRenderEffect()
{}
void PostRenderEffect()
{
WriteText("MADRIX " +GetSoftwareVersion());
}
(Description)
SetText (SCE Ticker) and GetTime
Copy and paste the script into the Macro Editor of the effect SCE Ticker and monitor the Preview Windows to see the result.
@scriptname="local ticker time";
@author="jky";
@version="MADRIX 2.10";
@description="Set time in SCE_Ticker text with GetTime and pm & am";
void InitEffect()
{}
void PreRenderEffect()
{
time t=GetTime();
string m,s;
if(t.min<10)
m="0"+(string)t.min;
else
m=(string)t.min;
if(t.sec<10)
s="0"+(string)t.sec;
else
s=(string)t.sec;
if(t.hour>12)
SetText((string)(t.hour-12)+":"+m+":"+s+" pm");
else
SetText((string) t.hour +":"+m+":"+s+" am");
}
void PostRenderEffect()
{}
void MatrixSizeChanged()
{
InitEffect();
}
(Description)
DrawPixelText - Font Size
Draws the text "Hello", which constantly increases over and over again.
@scriptname="";
@author="";
@version="";
@description="";
font f={10,
0,
0,
0,
FONT_WEIGHT_BOLD,
0,
0,
0,
CHARSET_DEFAULT,
PRECIS_OUT_DEFAULT,
PRECIS_CLIP_DEFAULT,
QUALITY_DEFAULT,
PITCH_DEFAULT,
FONT_FAMILY_SWISS,
"Arial"};
int i=0;
void InitEffect()
{
i=0;
}
void PreRenderEffect()
{
}
void PostRenderEffect()
{
i++;
f.height=i%40;
DrawPixelText(WHITE,f,"Hello",0,0,ROTATION_TEXT_NONE);
}
void MatrixSizeChanged()
{
InitEffect();
}
(Description)
DrawPixelText - Font Color
Draws the text "Hello" and changes its color.
@scriptname="";
@author="";
@version="";
@description="";
font f={20,
0,
0,
0,
FONT_WEIGHT_BOLD,
0,
0,
0,
CHARSET_DEFAULT,
PRECIS_OUT_DEFAULT,
PRECIS_CLIP_DEFAULT,
QUALITY_DEFAULT,
PITCH_DEFAULT,
FONT_FAMILY_SWISS,
"Arial"};
int i=0;
color col;
void InitEffect()
{
i=0;
col=WHITE;
}
void PreRenderEffect()
{
}
void PostRenderEffect()
{
i++;
col.r=i%255;
col.g=(i%512)/2;
col.b=(i%767)/3;
DrawPixelText(col,f,"Hello",0,0,ROTATION_TEXT_NONE);
}
void MatrixSizeChanged()
{
InitEffect();
}
(Description)
DrawPixelText - Moving Text
Draws the text "Hello", which moves from the upper left to the lower right.
@scriptname="";
@author="";
@version="";
@description="";
font f={20,
0,
0,
0,
FONT_WEIGHT_BOLD,
0,
0,
0,
CHARSET_DEFAULT,
PRECIS_OUT_DEFAULT,
PRECIS_CLIP_DEFAULT,
QUALITY_DEFAULT,
PITCH_DEFAULT,
FONT_FAMILY_SWISS,
"Arial"};
int i=0;
void InitEffect()
{
i=0;
}
void PreRenderEffect()
{
}
void PostRenderEffect()
{
i++;
i=i%50;
DrawPixelText(RED,f,"Hello",i,i,ROTATION_TEXT_NONE);
}
void MatrixSizeChanged()
{
InitEffect();
}
(Description)
DrawPixelText - Rotating Text
Draws the text "Hello", which rotates by 90°, 180°, and 270°.
@scriptname="";
@author="";
@version="";
@description="";
font f={20,
0,
0,
0,
FONT_WEIGHT_BOLD,
0,
0,
0,
CHARSET_DEFAULT,
PRECIS_OUT_DEFAULT,
PRECIS_CLIP_DEFAULT,
QUALITY_DEFAULT,
PITCH_DEFAULT,
FONT_FAMILY_SWISS,
"Arial"};
int i=0;
void InitEffect()
{
i=0;
}
void PreRenderEffect()
{
}
void PostRenderEffect()
{
i++;
i=i%400;
switch(i/100)
{
case 0:DrawPixelText(RED,f,"Hello",25,25,ROTATION_TEXT_NONE);break;
case 1:DrawPixelText(RED,f,"Hello",25,25,ROTATION_TEXT_90);break;
case 2:DrawPixelText(RED,f,"Hello",25,25,ROTATION_TEXT_180);break;
case 3:DrawPixelText(RED,f,"Hello",25,25,ROTATION_TEXT_270);break;
}
}
void MatrixSizeChanged()
{
InitEffect();
}
(Description)
GetTimeCode
Retrieves the currently used Time Code. This Script works in all four Script locations.
@scriptname="GetTimeCode";
@author="jky";
@version="2.14a";
@description="Returns the currently used Time Code";
void InitEffect()
{
}
void PreRenderEffect()
{
}
void PostRenderEffect()
{
time TimeCode=GetTimeCode();
WriteText("Timecode: "+(string)TimeCode.hour+":"+(string)TimeCode.min+":"
+(string)TimeCode.sec);
}
void MatrixSizeChanged()
{
InitEffect();
}
(Description)
GetDmxIn
Uses incoming DMX-IN data to show colors on the LED matrix. This Script works in all four Script locations.
@scriptname="DmxInToColor";
@author="jky";
@version="1.0";
@description="Read DMX-IN data and makes to matrix color";
const int CHANNEL_START=0; // start by channel 1
const int CHANNEL_COUNT=3; // use this number of channels
const int UNIVERSE=0; // use this universe for DMX-IN data
int DmxValues[]; // field of DMX Universe
color col;
void InitEffect()
{
col=BLACK;
if(IsDmxInEnabled()==0)// if DMX-In is enabled
WriteText("DMX-IN is disabled!");
}
void RenderEffect()
{
if(IsDmxInEnabled()==1)// if DMX-In enabled?
{
// Get the DMX values from selected Universe
GetDmxIn(DmxValues,CHANNEL_START,CHANNEL_COUNT,UNIVERSE);
// set dmx value to color
col.r= DmxValues[0]; // channel 1 to red
col.g= DmxValues[1]; // channel 2 to green
col.b= DmxValues[2]; // channel 3 to blue
}
else
col=BLACK;// no DMX then no color
Clear(col);// set complete matrix with color
//Information for help
//WriteText("Set color with Value Red="+(string)col.r+", Green="+(string)col.g+",
Blue="+(string)col.b);
}
void MatrixSizeChanged()
{
InitEffect();
}
(Description)
GetMidiInNoteValue and GetMidiInControlValue
Uses incoming MIDI-IN data to control the Master Fader. This Script works in the Main Output Macro.
@scriptname="MIDItoMaster";
@author="jky";
@version="2.14b";
@description="Uses incoming MIDI to control the Master Fader";
const int NOTE=0; // MIDI Note for control
const int CHANNEL=0; // MIDI Channel for control
const int DEVICE_ID=0; // MIDI Device for control
void InitEffect()
{
}
void PreRenderEffect()
{
}
void PostRenderEffect()
{
if(IsMidiInEnabled()==1)
{
const float Value = (float)GetMidiInNoteValue(NOTE,CHANNEL,DEVICE_ID)/127.0;
// MIDI NOTES 0x9, 0x8
//const float Value = (float)GetMidiInControlValue(NOTE,CHANNEL,DEVICE_ID)/127.0;
// MIDI CONTROLLER 0xb
SetMasterFader(Value*255);
}
}
void MatrixSizeChanged()
{
InitEffect();
}
(Description)
GetMidiInNote and GetMidiInControl
Uses incoming MIDI-IN data to control the Master Fader and the Audio Level. This Script works in the Main Output Macro.
@scriptname="MIDItoMasterandAudio";
@author="jky";
@version="2.14b";
@description="Uses incoming MIDI of 2 channels to control the Master Fader and Audio Level";
const int NOTE=0; // MIDI Note for control
const int NOTE_COUNT=2; // MIDI Note Count for control
const int CHANNEL=0; // MIDI Channel for control
const int DEVICE_ID=0; // MIDI Device for control
int MidiData[];
void InitEffect()
{
}
void PreRenderEffect()
{
}
void PostRenderEffect()
{
if(IsMidiInEnabled()==1)
{
GetMidiInNote(MidiData, NOTE, NOTE_COUNT, CHANNEL, DEVICE_ID);
// MIDI NOTES 0x9, 0x8
//GetMidiInControl(MidiData, NOTE, NOTE_COUNT, CHANNEL, DEVICE_ID);
// MIDI NOTES 0xb
SetMasterFader(MidiData[0]*255/127);
SetAudioFader(MidiData[1]*255/127);
}
}
void MatrixSizeChanged()
{
InitEffect();
}
(Description)
Installed Examples
Throughout this MADRIX Script Help and Manual a lot of practical script examples are already given.
If you would like to see some more examples, several exemplary scripts are already installed on your PC if you have enabled this option during the installation process.
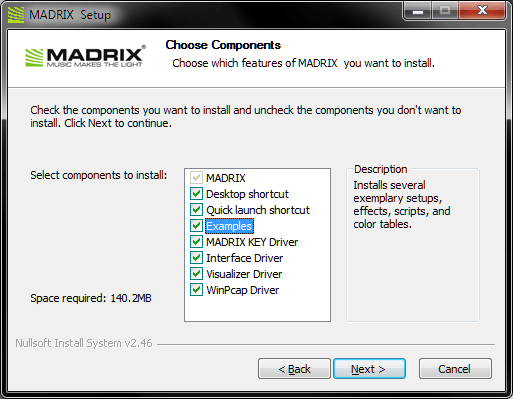
You can find the examples on your hard disk. Please navigate to C:\Users\USERNAME\Documents\MADRIX\scripts.
(Please choose your Windows username for USERNAME).
The folder contains a link "Samples". This link will take you to another folder that contains various examples.
|